In this tutorial, you shall learn how to copy a file to a new path in Kotlin using copyTo() function, with example programs.
Kotlin – Copy file
To copy a file in Kotlin, you can use the copyTo() function of java.io.File class.
Steps to copy file
- Consider that we need to copy a file from one location (source) to another location (target).
- Create two file objects: one with the source file path, and the other with target file path, using java.io.File class.
- Call copyTo() function on the source file object, and pass the target file object as argument. The function copies the source file to target file.
- The copyTo() function returns the target file object.
val sourceFile = File("source/file") val targetFile = File("target/file") sourceFile.copyTo(targetFile)
ADVERTISEMENT
Example
For this example, we will consider a file named info.txt at the path mydata/set1/info.txt
.
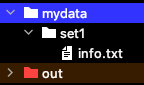
In the following program, we copy the file mydata/set1/info.txt
to mydata/set2/info.txt
.
If any directories in the target path are missing, those directories are created.
Main.kt
import java.io.File fun main() { val sourceFilePath = "mydata/set1/info.txt" val targetFilePath = "mydata/set2/info.txt" val sourceFile = File(sourceFilePath) val targetFile = File(targetFilePath) try { sourceFile.copyTo(targetFile) println("$sourceFile is copied to $targetFile") } catch (e: Exception) { println("Error occurred while copying file.") e.printStackTrace() } }
Output
mydata/set1/info.txt is copied to mydata/set2/info.txt
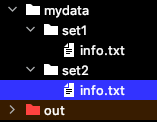
Conclusion
In this Kotlin Tutorial, we learned how to copy a file from one location to another using File.copyTo() function.