In this tutorial, you shall learn how to rename a file in Kotlin identified by a given path, using File.renameTo() function, with example programs.
Kotlin – Rename a File
To rename a file in Kotlin, you can use renameTo() function of java.io.File class.
Steps to rename a file
- Consider that we have a file (source) given by a path, and have to rename this file to a new name (target).
- Create two file objects: one for the source file, and one for the target file, with respective paths.
- Call renameTo() function on the source file and pass the target file as argument. The function returns true if renaming is successful, else it returns false.
</>
Copy
val sourceFile = File("path/to/source")
val targetFile = File("path/to/target")
sourceFile.renameTo(targetFile)
Example
Consider that we have a file named info.txt
, and we would like to rename it to info_1.txt
.
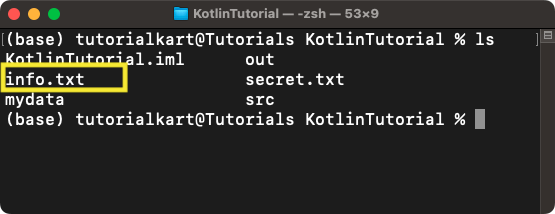
In the following program, we shall rename the file info.txt
to info_1.txt
by using File.renameTo() function.
Main.kt
</>
Copy
import java.io.File
fun main() {
val sourceFile = File("info.txt")
val targetFile = File("info_1.txt")
if ( sourceFile.renameTo(targetFile) ) {
println("File renamed successfully.")
} else {
println("Could not rename file.")
}
}
Output
File renamed successfully.
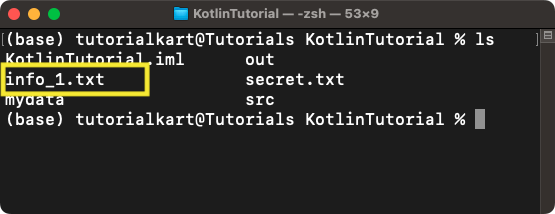
Reference tutorials for the above program
Conclusion
In this Kotlin Tutorial, we learned how to rename a file using java.io.File.renameTo() function.