Android ProgressBar (Determinate mode) – Kotlin Example
Android ProgressBar – Kotlin Example : In this Android Tutorial, we shall learn to indicate the progress of an operation using ProgressBar. We shall use an Example Android Application with dummy operation whose progress has to indicated to user.
ProgressBar could be used both Determinate and Indeterminate progress of a task.
- Indeterminate ProgressBar is used when you cannot estimate or track the progress of a task. By default ProgressBar is in Indeterminate mode.
- Determinate ProgressBar is used when you can estimate or track the progress of a task. To make ProgressBar determinate, add progress property, android:progress=”0″ , in the layout file for ProgressBar View.
In this tutorial, we shall see an example for Determinate ProgressBar. In our next tutorial, we shall learn Android Indeterminate ProgressBar
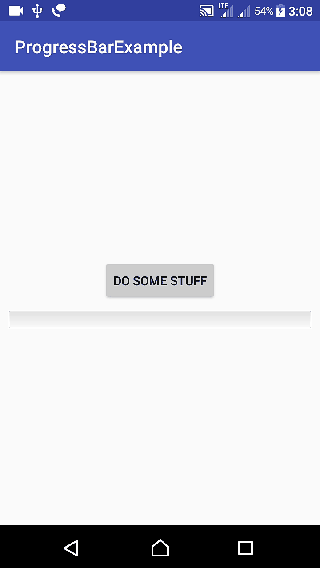
Progress of ProgressBar can be set using progress property as shown below :
progressBar.progress = 55
The value provided for the property is the percentage. If 55 is assigned to progress, then 55% of the ProgressBar would be marked with progress.
Following are the details of the Android Application we created for this example.
Application Name | ProgressBarExample |
Company name | tutorialkart.com |
Minimum SDK | API 21: Android 5.0 (Lollipop) |
Activity | Empty Activity |
Create an Android Application with Kotlin Support with above details and keeping rest to default. Replace activity_main.xml and MainActivity.kt with the following content.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center"
tools:context="com.tutorialkart.progressbarexample.MainActivity">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="116dp"
android:text="Do some stuff" />
<ProgressBar
style="@android:style/Widget.ProgressBar.Horizontal"
android:id="@+id/progressBar1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:progress="0"/>
</LinearLayout>
MainActivity.kt
package com.tutorialkart.progressbarexample
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
private var progressBarStatus = 0
var dummy:Int = 0
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the references from layout file
var btnStartProgress = this.button1
var progressBar = this.progressBar1
// when button is clicked, start the task
btnStartProgress.setOnClickListener { v ->
// task is run on a thread
Thread(Runnable {
// dummy thread mimicking some operation whose progress can be tracked
while (progressBarStatus < 100) {
// performing some dummy operation
try {
dummy = dummy+25
Thread.sleep(1000)
} catch (e: InterruptedException) {
e.printStackTrace()
}
// tracking progress
progressBarStatus = dummy
// Updating the progress bar
progressBar.progress = progressBarStatus
}
}).start()
}
}
}