Kotiln Android – Login Form
In this tutorial, we shall learn how to implement a Login Form in an Android Activity with the help of an Example Android Application.
The Login Form shall include two edit text views for user name and password. There shall be a reset button to reset the fields and a submit button to read the values for user name and password and further process them. OnClickListeners are setup for reset and submit buttons.
A mock-up screenshot of the Login Form Example in Kotlin Android would be as shown in the following.
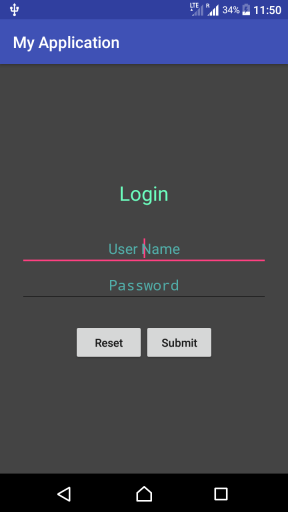
Example – Kotlin Android Login Screen
Create an Android Application with Kotlin Support and replace activity_main.xml and MainActivity.kt with the following content, which will help you create a Login Form.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.tutorialkart.myapplication.MainActivity">
<LinearLayout
android:id="@+id/ll_main_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:background="#444444"
android:padding="25dp"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="25dp"
android:textColor="#6dffbf"
android:padding="30dp"
android:text="Login"/>
<EditText
android:id="@+id/et_user_name"
android:hint="User Name"
android:textColor="#6bfff7"
android:textColorHint="#52afaa"
android:textAlignment="center"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/et_password"
android:hint="Password"
android:textColor="#6bfff7"
android:textColorHint="#52afaa"
android:textAlignment="center"
android:inputType="textPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="25dp"
android:orientation="horizontal">
<Button
android:id="@+id/btn_reset"
android:text="Reset"
android:textAllCaps="false"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn_submit"
android:text="Submit"
android:textAllCaps="false"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
</LinearLayout>
</android.support.constraint.ConstraintLayout>
MainActivity.kt
package com.tutorialkart.myapplication
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import android.widget.*
/**
* A Login Form Example in Kotlin Android
*/
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get reference to all views
var et_user_name = findViewById(R.id.et_user_name) as EditText
var et_password = findViewById(R.id.et_password) as EditText
var btn_reset = findViewById(R.id.btn_reset) as Button
var btn_submit = findViewById(R.id.btn_submit) as Button
btn_reset.setOnClickListener {
// clearing user_name and password edit text views on reset button click
et_user_name.setText("")
et_password.setText("")
}
// set on-click listener
btn_submit.setOnClickListener {
val user_name = et_user_name.text;
val password = et_password.text;
Toast.makeText(this@MainActivity, user_name, Toast.LENGTH_LONG).show()
// your code to validate the user_name and password combination
// and verify the same
}
}
}
When you run this Android Application, you will get the following output.
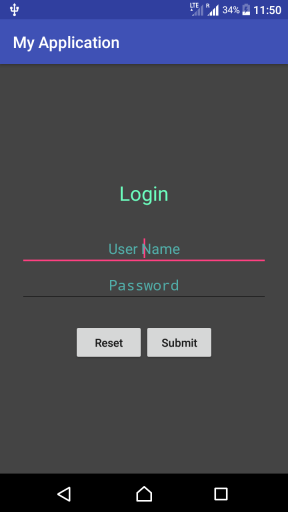
User would be able to enter user name and password.
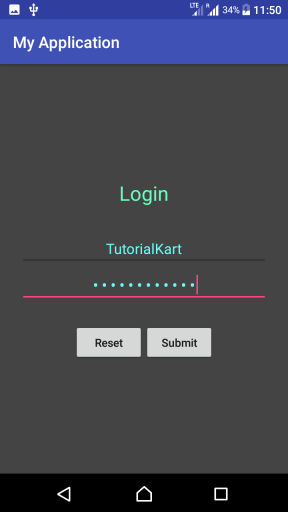
When he clicks on Submit, we are showing user name in Toast. But you may send it to your back end systems to validate the login credentials.
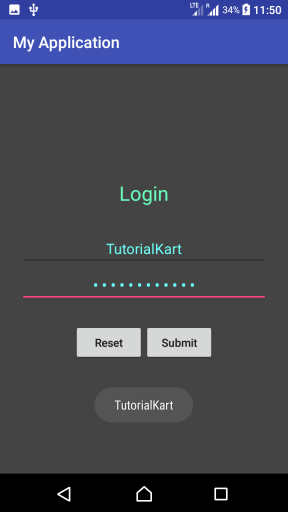
Conclusion
In this Kotlin Android Tutorial, we learned how to build a Login screen in Kotlin Android using basic widgets like TextView, EditText, Button, etc.