Toast in Android is used to display a piece of text for a short span of time. When Toast is made, the piece of text is appears on the screen, stays there on the screen for about 2 or 3 to 5 seconds and disappears.
In this tutorial, we will learn how to display Toast in an Android Application.
Code – Android Toast
A quick look into code snippets of Android Toast – Kotlin Examples
Toast.makeText(this, "Hi there! This is a Toast.", Toast.LENGTH_SHORT).show() Toast.makeText(this, "Hi there! This is a Toast.", Toast.LENGTH_LONG).show()
From the examples above, makeText method needs context, toast message and toast duration (LENGTH_SHORT or LENGTH_LONG). And finally the show() method displays the toast for the specified duration.
Example Android Application – Android Toast
Android Toast – Kotlin Example : In this Android Tutorial, we shall learn to make a Toast with example Android Applications.
We shall use following two tutorials in demonstrating Toast.
Example Android Application with Kotlin Support Create Activity with name ‘ToastActivity’.
Button OnclickListener On click of a button, we shall display the Toast. This scenario could be generalised as displaying a piece of text when an event occurs.
A typical toast is shown below :
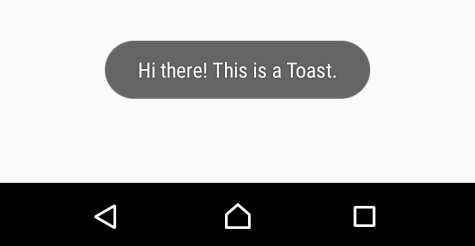
Following is the code for ToastActivity.kt and activity_toast.xml.
activity_toast.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.tutorialkart.myapplication.ToastActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:padding="25dp" android:orientation="horizontal"> <Button android:id="@+id/btn_click_me" android:background="@drawable/btn_round_edge" android:text="Click me for Toast" android:textAllCaps="false" android:padding="10dp" android:textSize="25dp" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout> </android.support.constraint.ConstraintLayout>
ToastActivity.kt
package com.tutorialkart.myapplication import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.Button import android.widget.Toast class ToastActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_toast) var btn_click_me = findViewById(R.id.btn_click_me) as Button btn_click_me.setOnClickListener { // make a toast on button click event Toast.makeText(this, "Hi there! This is a Toast.", Toast.LENGTH_LONG).show() } } }
When the application is built and run on an Android device,
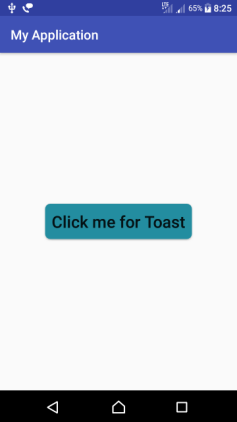
Click on the button, “Click me for Toast” to display the Toast
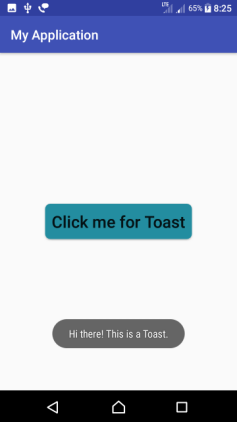
Try changing the Toast duration from Toast.LENGTH_LONG to Toast.LENGTH_SHORT and observe the display duration differences.
Conclusion
In this Kotlin Android Tutorial, we learned how to display a Toast upon some user interaction.