Kotlin Android SeekBar
Android SeekBar is kind of an extension to progress bar, with an addition functionality that user can touch the thumb and drag it to change the value.
In this tutorial, we will learn how to include a SeekBar in our main activity layout and read the values when user interacts with the SeekBar.
The following GIF shows how a user can touch the trackbar, and seek it to the left or right of the bar.
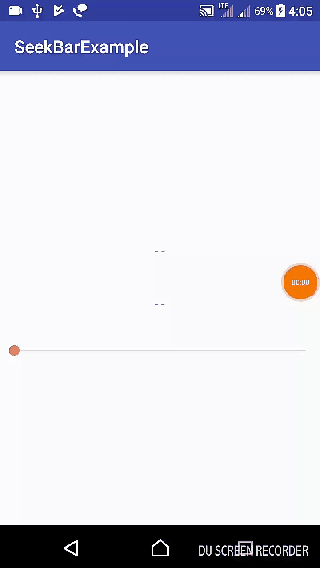
SeekBar Code
Following is a quick code snippet to use SeekBar in your layout file and Kotlin file respectively.
<SeekBar android:id="@+id/seekbar" android:layout_width="match_parent" android:padding="15dp" android:layout_height="wrap_content" />
import android.os.Bundle import android.widget.SeekBar import android.widget.SeekBar.OnSeekBarChangeListener import kotlinx.android.synthetic.main.activity_main.* class MainActivity : /** Other Classes, */OnSeekBarChangeListener { override fun onCreate(savedInstanceState: Bundle?) { this.seekbar!!.setOnSeekBarChangeListener(this) } override fun onProgressChanged(seekBar: SeekBar, progress: Int, fromUser: Boolean) { // called when progress is changed } override fun onStartTrackingTouch(seekBar: SeekBar) { // called when tracking the seekbar is started } override fun onStopTrackingTouch(seekBar: SeekBar) { // called when tracking the seekbar is stopped } }
By default, the range of progress is [0,100] in steps of 1.
Following is a step by step guide of what is happening in the above code snippet to use SeekBar.
Step 1: Create a SeekBar in layout file.
<SeekBar android:id="@+id/seekbarView" android:layout_width="match_parent" android:layout_height="wrap_content" />
Step 2: Add OnSeekBarChangeListener to the interface list of your Activity.
class MainActivity : /** Other Classes, */OnSeekBarChangeListener { }
Step 3: Set setOnSeekBarChangeListener to the SeekBar.
seekbarView!!.setOnSeekBarChangeListener(this)
Step 4: We have to override the following three methods of OnSeekBarChangeListener.
override fun onProgressChanged(seekBar: SeekBar, progress: Int, fromUser: Boolean) { // called when progress is changed } override fun onStartTrackingTouch(seekBar: SeekBar) { // called when tracking the seekbar is started } override fun onStopTrackingTouch(seekBar: SeekBar) { // called when tracking the seekbar is stopped }
Example – Android SeekBar
Following are the details of the Android Application we created for this example.
Application Name | SeekBarExample |
Company name | tutorialkart.com |
Minimum SDK | API 21: Android 5.0 (Lollipop) |
Activity | Empty Activity |
You may keep rest of the values as default and create Android Application with Kotlin Support.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.tutorialkart.seekbarexample.MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical"> <TextView android:id="@+id/progress" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="- -" android:padding="20dp"/> <TextView android:id="@+id/seekbarStatus" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="- -" android:padding="20dp"/> <SeekBar android:id="@+id/seekbar" android:layout_width="match_parent" android:padding="15dp" android:layout_height="wrap_content" /> </LinearLayout> </android.support.constraint.ConstraintLayout>
MainActivity.kt
package com.tutorialkart.seekbarexample import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.SeekBar import android.widget.SeekBar.OnSeekBarChangeListener import android.widget.TextView import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity(),OnSeekBarChangeListener { private var progressView: TextView? = null private var seekbarStatusView: TextView? = null private var seekbarView: SeekBar? = null override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) progressView = this.progress seekbarStatusView = this.seekbarStatus seekbarView = this.seekbar seekbarView!!.setOnSeekBarChangeListener(this) } override fun onProgressChanged(seekBar: SeekBar, progress: Int, fromUser: Boolean) { progressView!!.text = progress.toString() seekbarStatusView!!.text = "Tracking Touch" } override fun onStartTrackingTouch(seekBar: SeekBar) { seekbarStatusView!!.text = "Started Tracking Touch" } override fun onStopTrackingTouch(seekBar: SeekBar) { seekbarStatusView!!.text = "Stopped Tracking Touch" } }
Output
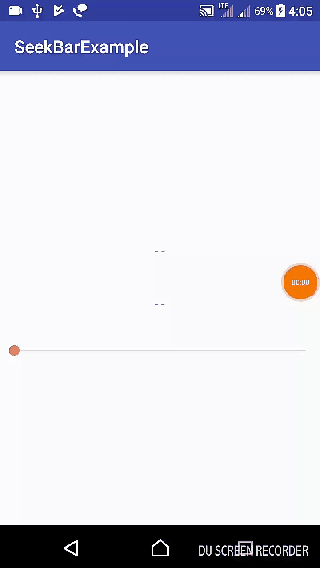
Conclusion
In this Android Tutorial : Android SeekBar – Kotlin Example, we have learnt to use SeekBar with an example Android Application.