Add a Cookie
To add a cookie using Selenium for Java, call manage().addCookie()
on the WebDriver object and pass the Cookie object as argument to the addCookie()
method.
The following is a simple code snippet to add a cookie named "dummy"
with value "xyz123"
.
</>
Copy
driver.manage().addCookie(new Cookie("dummy", "xyz123"));
where driver
is a WebDriver object. Cookie() constructor can take the key and value as arguments to create a cookie object.
This method call returns void.
Example
In the following program, we write Selenium Java code to visit Wikipedia Home Page, and add the Cookie with key-value pair (“dummy”, “xyz123”).
Java Program
</>
Copy
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
try {
driver.get("https://en.wikipedia.org/wiki/Main_Page");
driver.manage().addCookie(new Cookie("dummy", "xyz123"));
System.out.println("Cookies : \n" + driver.manage().getCookies());
} finally {
driver.quit();
}
}
}
Output
Cookies :
[dummy=xyz123; path=/; domain=en.wikipedia.org;secure;, GeoIP=IN:TG:Hyderabad:17.43:78.51:v4; path=/; domain=.wikipedia.org;secure;, WMF-Last-Access-Global=26-Jun-2022; expires=Thu, 28 Jul 2022 09:30:20 IST; path=/; domain=.wikipedia.org;secure;, WMF-Last-Access=26-Jun-2022; expires=Thu, 28 Jul 2022 09:30:20 IST; path=/; domain=en.wikipedia.org;secure;]
Screenshot
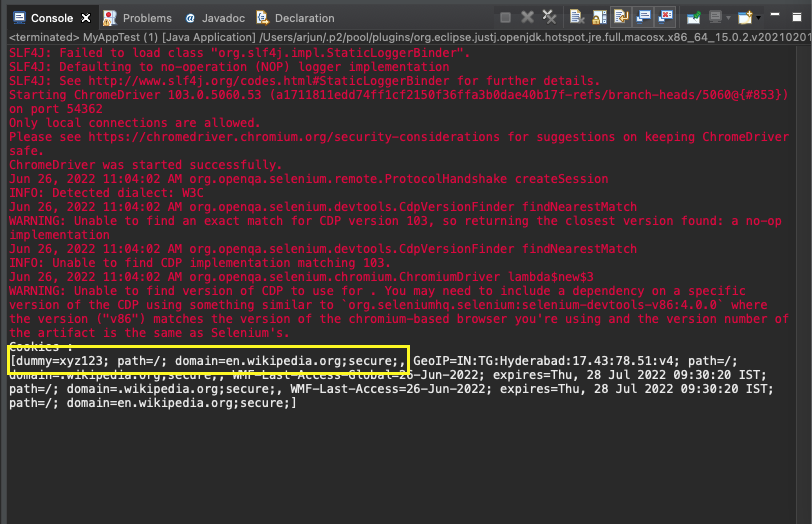
As you may notice, other values in the cookie like path and domain are set to default values for the cookie.