Click a Link based on Link Text
To click a link (anchor element) based on link text using Selenium in Java, find the element (web element) by link text By.linkText()
and then call click()
function on this web element.
The following is a simple code snippet where we find the link with the text "About"
and then click on this link using Selenium.
WebElement aboutLink = driver.findElement(By.linkText("About")); aboutLink.click();
Example
ADVERTISEMENT
In the following, we write Selenium Java program to visit google.com, and click the link with text "About"
.
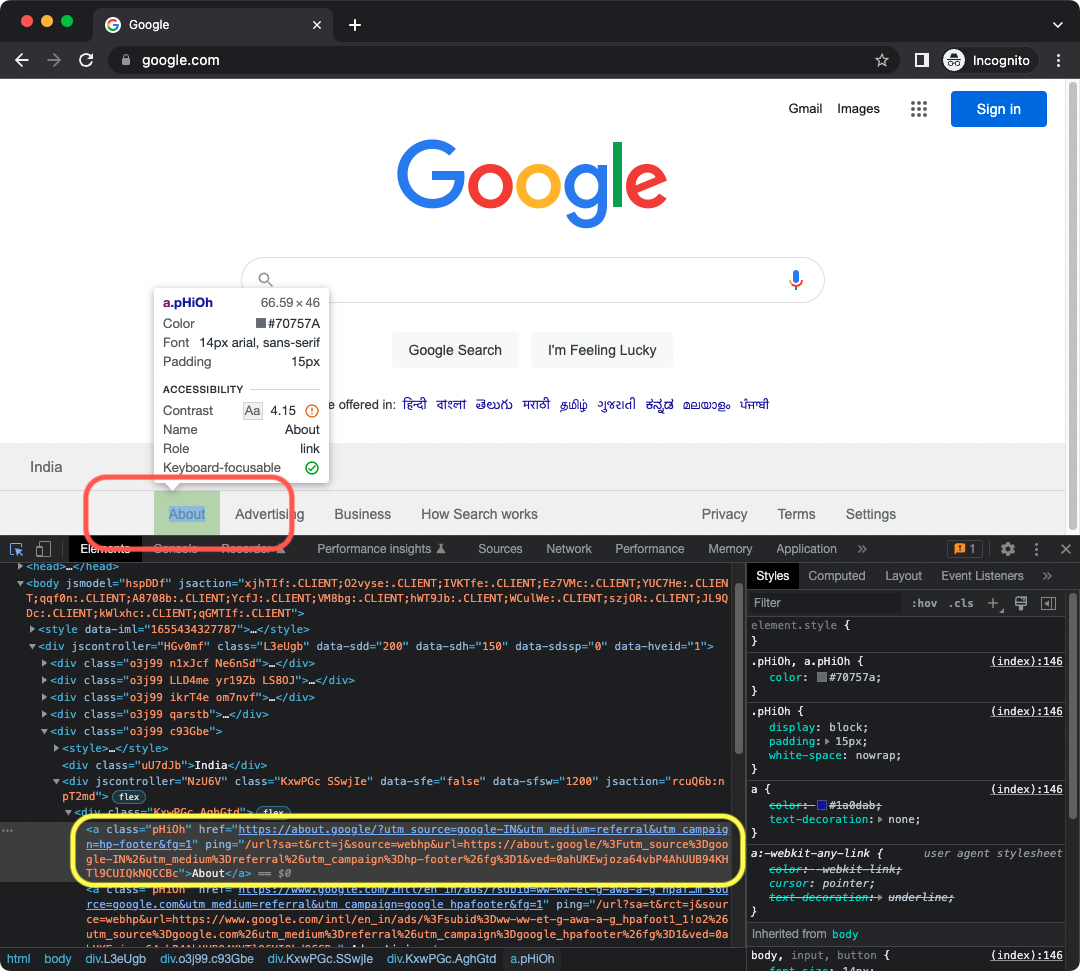
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr"); WebElement aboutLink = driver.findElement(By.linkText("About")); aboutLink.click(); driver.quit(); } }
Screenshots
1. Initialize web driver and visit google.com.
WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr");
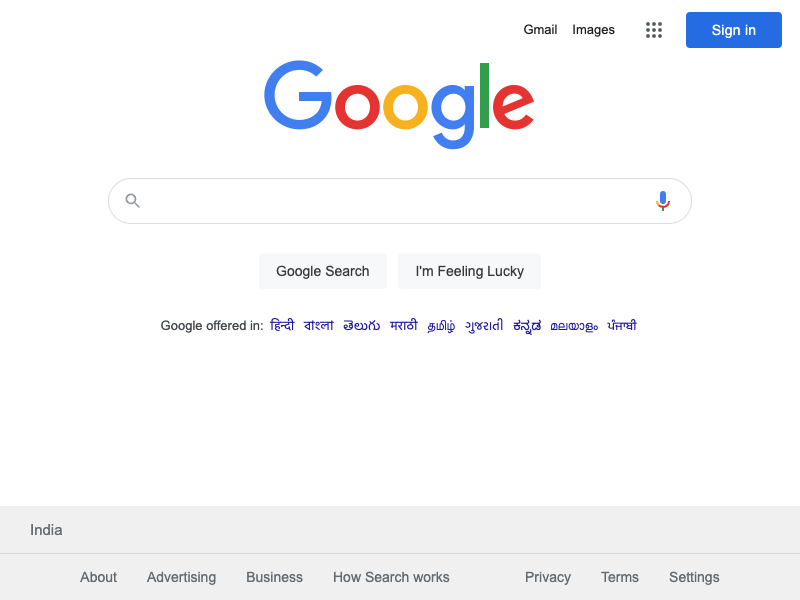
2. Find Web Element using link text “About”, and click on the element.
WebElement aboutLink = driver.findElement(By.linkText("About")); aboutLink.click();
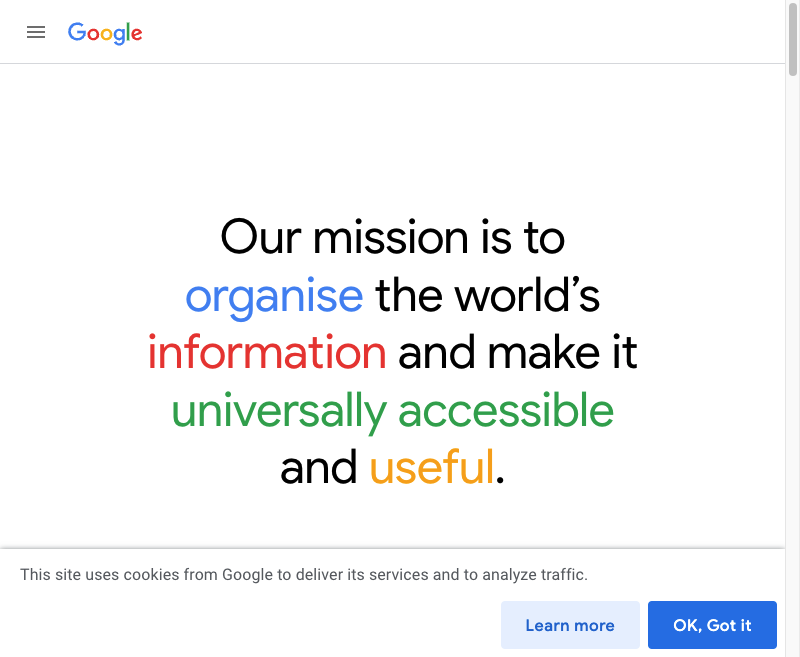