Get Previous Sibling Web Element
To get the immediate previous sibling element of given web element using Selenium for Java, find elements on the given web element by XPath with the XPath expression as "preceding-sibling::*"
, and get the last element in the list.
The following is a simple code snippet to get all the previous sibling elements of the web element element
.
List<WebElement> allPreviousSiblings = element.findElements(By.xpath("preceding-sibling::*"))
Next, we have to get the last element in the list, which would be the immediate previous sibling.
WebElement previousSibling = allPreviousSiblings.get(allPreviousSiblings.size() - 1);
Example
In the following program, we write Selenium Java code to visit Tutorialkart Home Page, and find the immediate previous sibling element of the link element <a href="/python/">Python</a>
. We shall print the outer HTML attribute of both the element and previous sibling element to standard output.
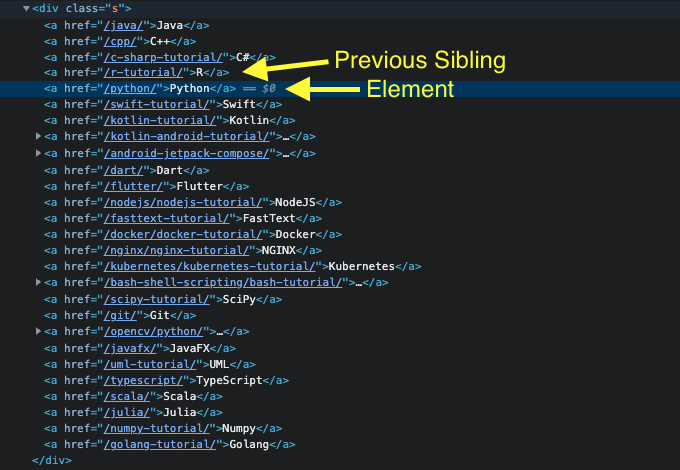
Java Program
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialkart.com/"); //child element WebElement element = driver.findElement(By.xpath("//a[@href=\"/python/\"]")); System.out.println("Child\n" + element.getAttribute("outerHTML")); //get previous sibling element List<WebElement> allPreviousSiblings = element.findElements(By.xpath("preceding-sibling::*")); WebElement previousSibling = allPreviousSiblings.get(allPreviousSiblings.size() - 1); System.out.println("\n\nPrevious Sibling\n" + previousSibling.getAttribute("outerHTML")); driver.quit(); } }
Output
Child <a href="/python/">Python</a> Previous Sibling <a href="/r-tutorial/">R</a>
Screenshot
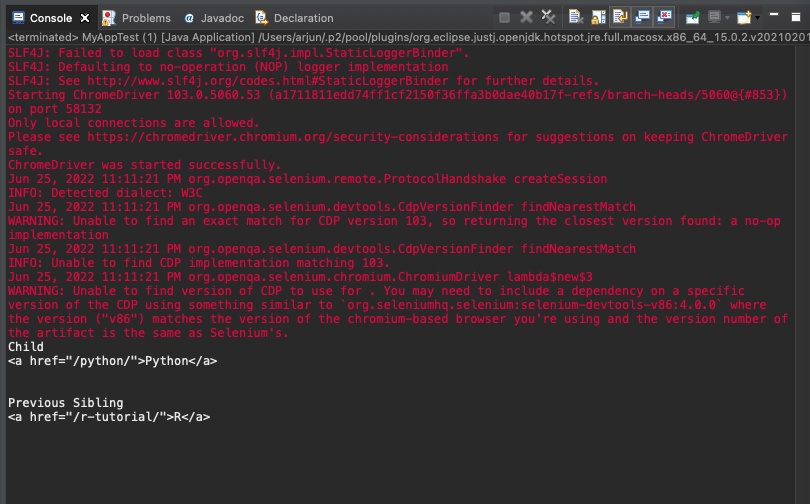