Delete All Cookies
To delete all the cookies for current browsing context using Selenium for Java, call manage().deleteAllCookies()
on the WebDriver object.
The following is a simple code snippet to get all the cookies
driver.manage().deleteAllCookies()
This expression returns void.
ADVERTISEMENT
Note: Please note that the server may set cookies during page load. We shall cover an example, to explicitly wait for specific period of time after hitting the URL, and then delete the cookies.
Example
In the following program, we write Selenium Java code to visit Wikipedia Home Page, and delete all the Cookies.
Java Program
import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://en.wikipedia.org/wiki/Main_Page"); //delete all cookies driver.manage().deleteAllCookies();; } finally { driver.quit(); } } }
To check if the cookies are deleted, let us print the cookies before and after deleting the cookies. Also, we shall wait for 5 seconds after deleting the cookies
Java Program
import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://en.wikipedia.org/wiki/Main_Page"); wait(driver); System.out.println("Before Deleting Cookies : \n" + driver.manage().getCookies()); //delete all cookies driver.manage().deleteAllCookies(); System.out.println("\nAfter Deleting Cookies: \n" + driver.manage().getCookies()); } finally { driver.quit(); } } public static void wait(WebDriver driver) { synchronized (driver) { try { driver.wait(5000); } catch (InterruptedException e) { e.printStackTrace(); } } } }
Output
Before Deleting Cookies : [enwikimwuser-sessionId=0cb2b2cad618ffbd9bd4; path=/; domain=en.wikipedia.org, GeoIP=IN:TG:Hyderabad:17.43:78.51:v4; path=/; domain=.wikipedia.org;secure;, enwikiwmE-sessionTickLastTickTime=1656217923121; expires=Tue, 26 Jul 2022 10:02:03 IST; path=/; domain=en.wikipedia.org, WMF-Last-Access-Global=26-Jun-2022; expires=Thu, 28 Jul 2022 08:28:19 IST; path=/; domain=.wikipedia.org;secure;, enwikiwmE-sessionTickTickCount=1; expires=Tue, 26 Jul 2022 10:02:03 IST; path=/; domain=en.wikipedia.org, WMF-Last-Access=26-Jun-2022; expires=Thu, 28 Jul 2022 08:28:19 IST; path=/; domain=en.wikipedia.org;secure;] After Deleting Cookies: []
Screenshot
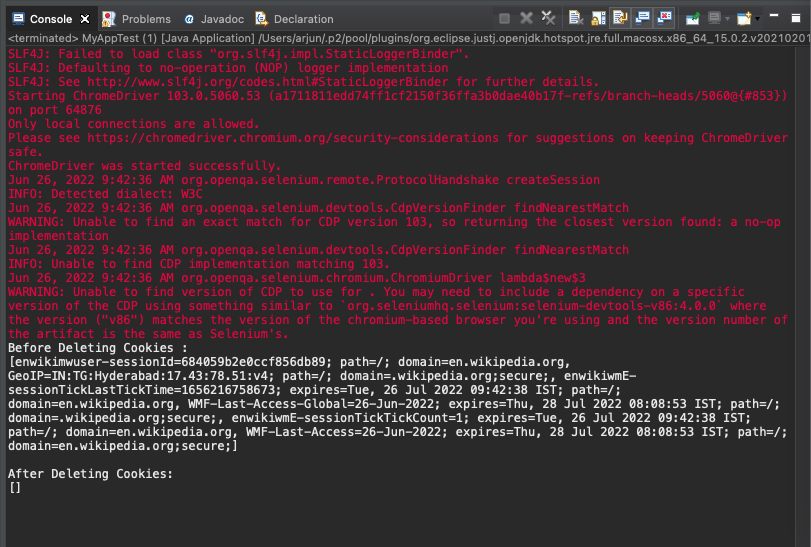