Execute JavaScript using Selenium
To execute custom JavaScript on web page using Selenium in Java, we have to use Selenium’s JavaScriptExecutor class.
JavaScriptExecutor.executeScript() takes the JavaScript code as String via first argument. In the second argument, we may pass optional arguments for the JavaScript code.
The following is a simple code snippet to execute JavaScript using WebDriver object driver
, to get the pageYOffset value of browser window.
JavascriptExecutor jsExecutor = (JavascriptExecutor)driver; jsExecutor.executeScript("return window.pageYOffset;")
The following code snippet shows how to pass arguments to JavaScript code.
WebElement footer = driver.findElement(By.id("footer")); JavascriptExecutor jsExecutor = (JavascriptExecutor)driver; jsExecutor.executeScript("arguments[0].scrollIntoView(true);", footer);
Here, we are passing the web element as argument, and inside the JavaScript we are accessing this web element using arguments array.
Example
In the following program, we write Selenium Java code to visit WikiPedia Main Page, and execute some JavaScript on this webpage.
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://en.wikipedia.org/wiki/Main_Page"); //scroll to footer WebElement footer = driver.findElement(By.id("footer")); JavascriptExecutor jsExecutor = (JavascriptExecutor)driver; jsExecutor.executeScript("arguments[0].scrollIntoView(true);", footer); //get scroll position Long scrollPosition = (Long) ((JavascriptExecutor) driver).executeScript("return window.pageYOffset;"); System.out.println("Scroll Position : " + scrollPosition); driver.quit(); } }
Output
Scroll Position : 2212
Screenshot
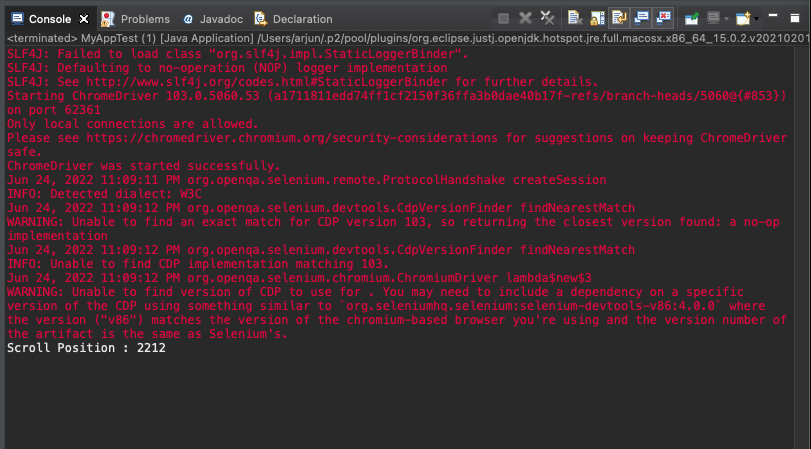