Get Scroll Position of Web Page
To get the scroll position of web page using Selenium in Java, we have to use JavaScript to get the page offset along Y-axis, and execute this JavaScript code from Selenium JavascriptExecutor.
The following is the code snippet to get page offset / scroll position of web page along Y-axis.
return window.pageYOffset;
We have to run the above JavaScript code on the webpage using Selenium’s JavascriptExecutor.
JavascriptExecutor jsExecutor = (JavascriptExecutor)driver; jsExecutor.executeScript("return window.pageYOffset;")
where driver
is the WebDriver object.
The above statement returns a Java object, and we have to cast this object to Long datatype.
Example
In the following program, we write Selenium Java code to visit WikiPedia Main Page, and print the scroll position of the web page.
Java Program
import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://en.wikipedia.org/wiki/Main_Page"); JavascriptExecutor jsExecutor = (JavascriptExecutor)driver; Long scrollPosition = (Long) jsExecutor.executeScript("return window.pageYOffset;"); System.out.println("Scroll Position : " + scrollPosition); driver.quit(); } }
Output
Scroll Position : 0
Screenshot
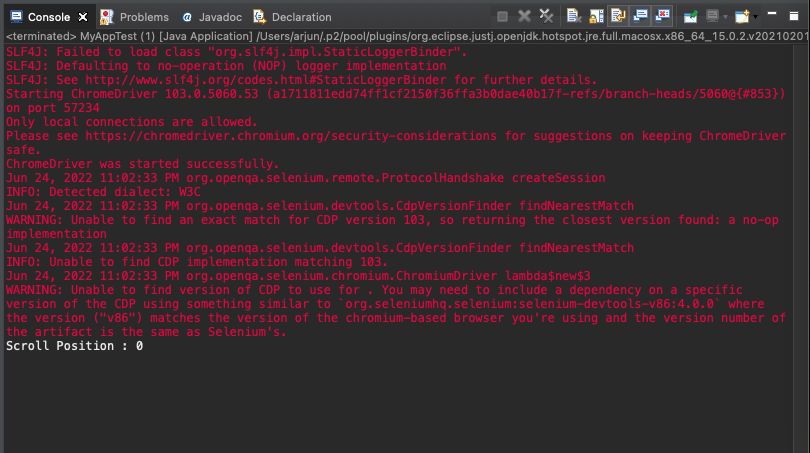
Since, we have not scrolled the web page, the scroll position or page offset along Y-axis is returned 0. We can scroll the page using JavaScript, and check if the scroll position returned from above code is same.
We shall modify the above program, to scroll the page to footer (id=footer), before getting the scroll position.
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://en.wikipedia.org/wiki/Main_Page"); //scroll to footer WebElement footer = driver.findElement(By.id("footer")); ((JavascriptExecutor)driver).executeScript("arguments[0].scrollIntoView(true);", footer); //get scroll position JavascriptExecutor jsExecutor = (JavascriptExecutor)driver; Long scrollPosition = (Long) jsExecutor.executeScript("return window.pageYOffset;"); System.out.println("Scroll Position : " + scrollPosition); driver.quit(); } }
Output
Scroll Position : 2212
Screenshot
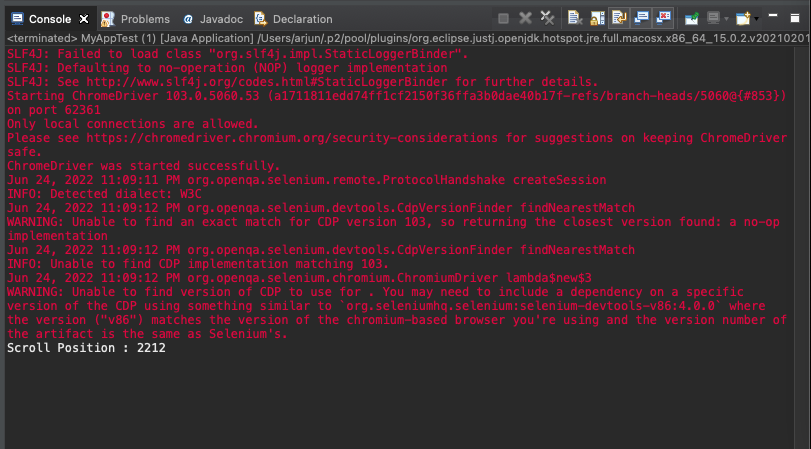