Find Element(s) by Attribute
To find the element(s) by the value of an attribute using Selenium in Java, use WebDriver.findElement(By.xpath())
or WebDriver.findElements(By.xpath())
and pass the expression with the attribute and value as argument to the xpath()
.
The following is a simple code snippet to get the first element whose attribute x has a value of y
WebElement element = driver.findElement(By.xpath("//*[@x='y']"));
WebDriver.findElement(By.xpath("//*[@x='y']"))
returns the a first web element whose attribute x
value is "y"
.
The following is a simple code snippet to get all the h2
elements
List<WebElement> elements = driver.findElements(By.xpath("//*[@x='y']"));
WebDriver.findElement(By.xpath("//*[@x='y']"))
returns the a list of all web elements in the web page, whose attribute x
‘s value is "y"
.
Example
Find First Web Element Based on Value of an Attribute
In the following, we write Selenium Java program to visit WikiPedia Main Page, then find the first web element with the class
attribute’s value of "extiw"
.
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://en.wikipedia.org/wiki/Main_Page"); WebElement element = driver.findElement(By.xpath("//*[@class='extiw']")); System.out.println(element.getText()); driver.quit(); } }
Screenshot
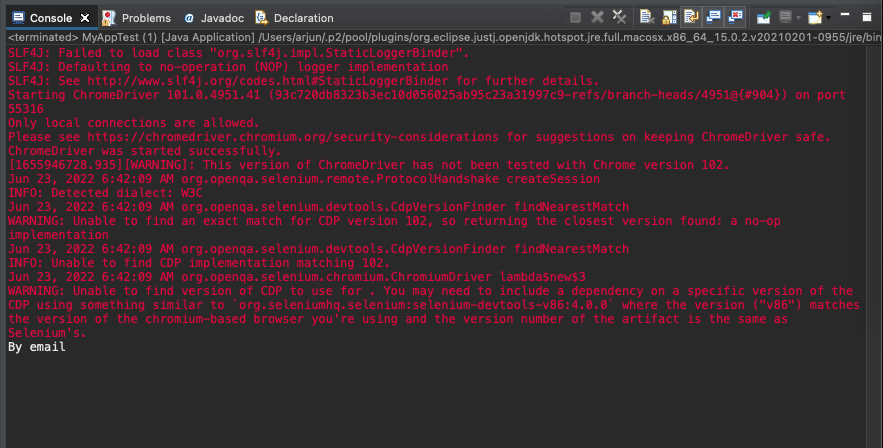
Find Web Elements Based on Value of an Attribute
In the following, we write Selenium Java program to visit WikiPedia Main Page, then find all the web elements with the class
attribute’s value of "extiw"
.
Java Program
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://en.wikipedia.org/wiki/Main_Page"); List<WebElement> elements = driver.findElements(By.xpath("//*[@class='extiw']")); for (WebElement element: elements) { System.out.println(element.getText()); } driver.quit(); } }
Screenshot
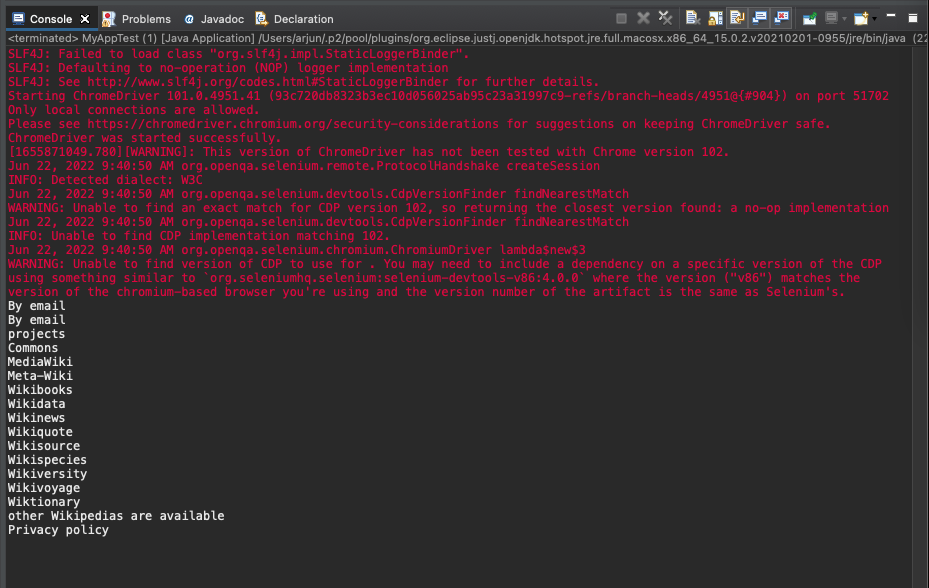