Get Current URL
To get the the current URL of web page programmatically using Selenium in Java, initialize a web driver and call getCurrentUrl()
method on the web driver object.
WebDriver.getCurrentUrl()
method returns a string representing the current URL that the browser is looking at.
The following is a simple code snippet to initialize a web driver and get the URL of the current web page.
WebDriver driver = new ChromeDriver(); //some code String currentUrl = driver.getCurrentUrl();
ADVERTISEMENT
Example
In the following program, we write Java code to initialize a web driver, visit google.com, search for the query 'tutorialkart'
, and get the current URL of that web page.
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr"); WebElement searchBox = driver.findElement(By.name("q")); searchBox.sendKeys("tutorialkart"); searchBox.sendKeys(Keys.RETURN); String currentUrl = driver.getCurrentUrl(); System.out.println(currentUrl); driver.quit(); } }
Screenshots
1. Initialize web driver and navigate to google.com.
WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr");
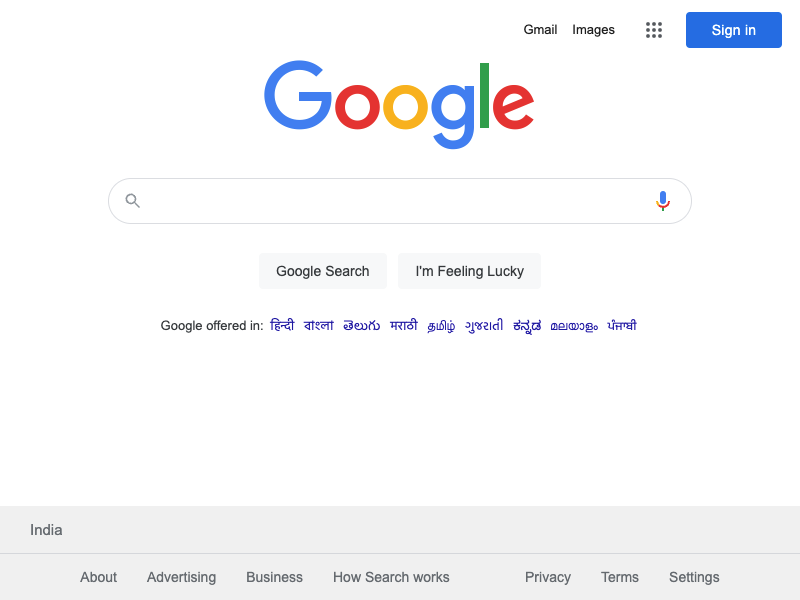
2. Enter search term “tutorialkart” in search box.
WebElement searchBox = driver.findElement(By.name("q")); searchBox.sendKeys("tutorialkart");
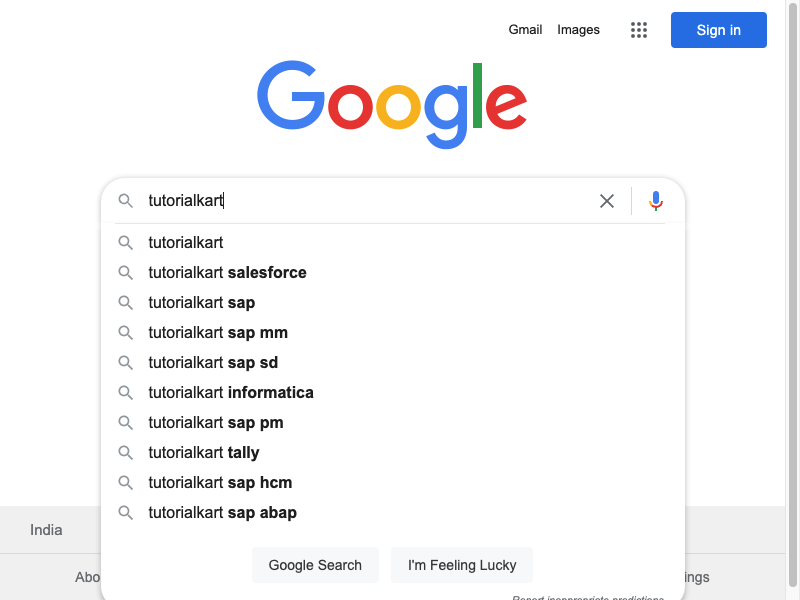
3. Enter RETURN key.
searchBox.sendKeys(Keys.RETURN);
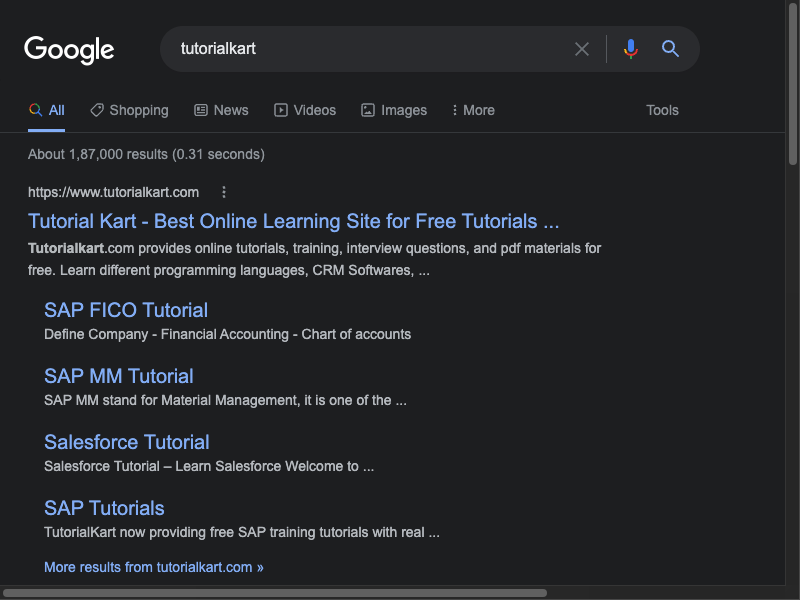
4. Get current URL.
String currentUrl = driver.getCurrentUrl(); System.out.println(currentUrl);
Console Output
https://www.google.com/search?q=tutorialkart&source=hp&ei=tT-YYvG9HJOs0QS5lZCwDg&iflsig=AJiK0e8AAAAAYphNxc186o-hjVXd2gF380pjnFL9RVu6&ved=0ahUKEwix7PqQ-o34AhUTVpQKHbkKBOYQ4dUDCAc&uact=5&oq=tutorialkart&gs_lcp=Cgdnd3Mtd2l6EAMyBQgAEIAEMgUIABCABDIFCAAQgAQyBQgAEIAEMgUIABCABDIFCAAQgAQyBQgAEIAEMgUIABCABDIFCAAQgAQyBQgAEIAEUABYlgFgiwtoAHAAeAGAAc8CiAHSCJIBBzAuMS4xLjKYAQCgAQE&sclient=gws-wiz