Delete Cookie by Name
To delete a cookie by name using Selenium for Java, call manage().deleteCookieNamed()
on the WebDriver object and pass the name of the cookie as argument to the deleteCookieNamed()
method.
The following is a simple code snippet to delete the cookie named “GeoIP”.
driver.manage().deleteCookieNamed("GeoIP")
where driver
is a WebDriver object.
ADVERTISEMENT
This method call returns void.
Example
In the following program, we write Selenium Java code to visit Wikipedia Home Page, and delete the Cookie named GeoIP.
Java Program
import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://en.wikipedia.org/wiki/Main_Page"); //wait for 5 seconds synchronized (driver) { driver.wait(5000); } System.out.println("Before Deleting Cookies : \n" + driver.manage().getCookies()); //delete cookie by name driver.manage().deleteCookieNamed("GeoIP"); System.out.println("\nAfter Deleting Cookies : \n" + driver.manage().getCookies()); } catch (InterruptedException e) { e.printStackTrace(); } finally { driver.quit(); } } }
Output
Before Deleting Cookies : [enwikimwuser-sessionId=c5ab2dc5145c6886f835; path=/; domain=en.wikipedia.org, GeoIP=IN:TG:Hyderabad:17.43:78.51:v4; path=/; domain=.wikipedia.org;secure;, enwikiwmE-sessionTickLastTickTime=1656219340714; expires=Tue, 26 Jul 2022 10:25:40 IST; path=/; domain=en.wikipedia.org, WMF-Last-Access-Global=26-Jun-2022; expires=Thu, 28 Jul 2022 08:51:57 IST; path=/; domain=.wikipedia.org;secure;, enwikiwmE-sessionTickTickCount=1; expires=Tue, 26 Jul 2022 10:25:40 IST; path=/; domain=en.wikipedia.org, WMF-Last-Access=26-Jun-2022; expires=Thu, 28 Jul 2022 08:51:57 IST; path=/; domain=en.wikipedia.org;secure;] After Deleting Cookies : [enwikimwuser-sessionId=c5ab2dc5145c6886f835; path=/; domain=en.wikipedia.org, enwikiwmE-sessionTickLastTickTime=1656219340714; expires=Tue, 26 Jul 2022 10:25:40 IST; path=/; domain=en.wikipedia.org, WMF-Last-Access-Global=26-Jun-2022; expires=Thu, 28 Jul 2022 08:51:57 IST; path=/; domain=.wikipedia.org;secure;, enwikiwmE-sessionTickTickCount=1; expires=Tue, 26 Jul 2022 10:25:40 IST; path=/; domain=en.wikipedia.org, WMF-Last-Access=26-Jun-2022; expires=Thu, 28 Jul 2022 08:51:57 IST; path=/; domain=en.wikipedia.org;secure;]
Screenshot
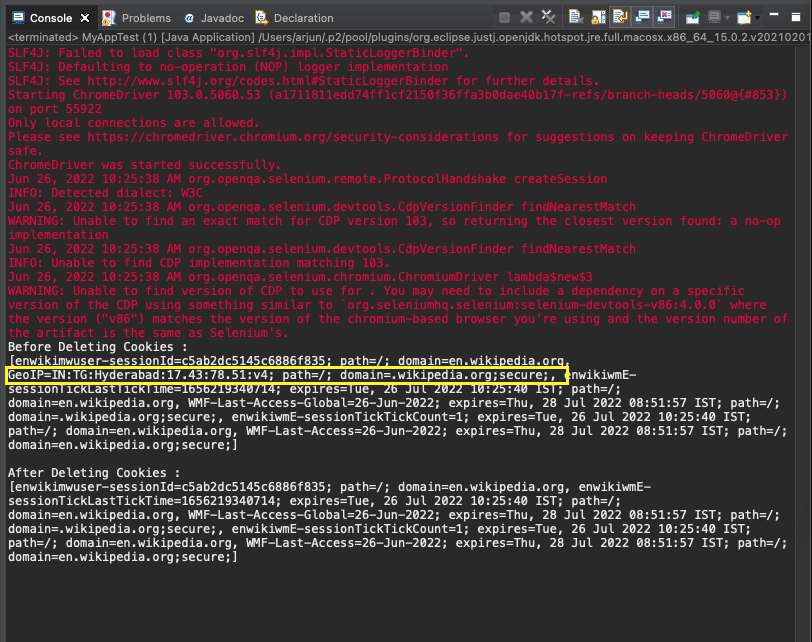