Get All Sibling Elements
To get all the sibling elements of a given web element using Selenium for Java,
- Find the following elements on the given web element by XPath with the XPath expression as
"following-sibling::*"
. - Find the preceding elements on the given web element by XPath with the XPath expression as
"preceding-sibling::*"
.
The following is a simple code snippet to get all the sibling elements of the web element element
.
element.findElements(By.xpath("following-sibling::*")) element.findElements(By.xpath("preceding-sibling::*"))
ADVERTISEMENT
These expressions returns List of WebElements. We may append these elements to get all the siblings in a single list.
Example
In the following program, we write Selenium Java code to visit Tutorialkart Home Page, and find all the sibling elements of the link element <a href="/java/">Java</a>
. We shall print the outer HTML attribute of both the element and sibling elements to standard output.
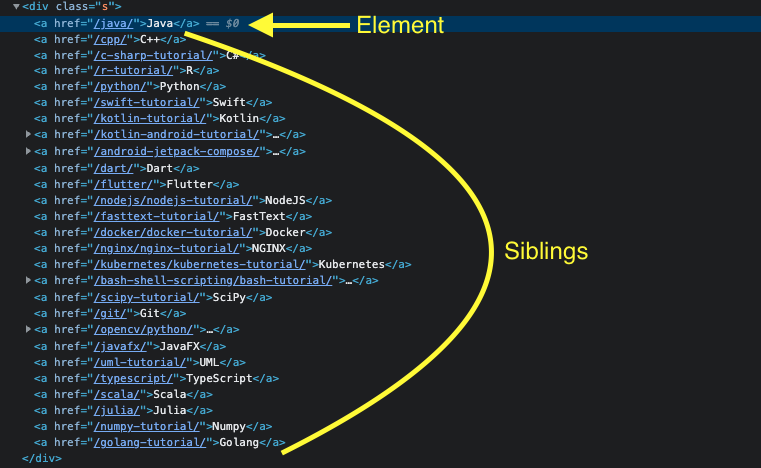
Java Program
import java.util.ArrayList; import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialkart.com/"); //child element WebElement element = driver.findElement(By.xpath("//a[@href=\"/java/\"]")); System.out.println("Element\n" + element.getAttribute("outerHTML")); //get all sibling elements List<WebElement> followingSiblings = element.findElements(By.xpath("following-sibling::*")); List<WebElement> precedingSiblings = element.findElements(By.xpath("preceding-sibling::*")); List<WebElement> siblings = new ArrayList(); siblings.addAll(followingSiblings); siblings.addAll(precedingSiblings); System.out.println("\nAll Siblings"); for(int i = 0; i < siblings.size(); i++) { System.out.println(siblings.get(i).getAttribute("outerHTML")); } driver.quit(); } }
Output
Element <a href="/java/">Java</a> All Siblings <a href="/cpp/">C++</a> <a href="/c-sharp-tutorial/">C#</a> <a href="/r-tutorial/">R</a> <a href="/python/">Python</a> <a href="/swift-tutorial/">Swift</a> <a href="/kotlin-tutorial/">Kotlin</a> <a href="/kotlin-android-tutorial/"><span>Kotlin Android</span></a> <a href="/android-jetpack-compose/"><span>Android Jetpack Compose</span></a> <a href="/dart/">Dart</a> <a href="/flutter/">Flutter</a> <a href="/nodejs/nodejs-tutorial/">NodeJS</a> <a href="/fasttext-tutorial/">FastText</a> <a href="/docker/docker-tutorial/">Docker</a> <a href="/nginx/nginx-tutorial/">NGINX</a> <a href="/kubernetes/kubernetes-tutorial/">Kubernetes</a> <a href="/bash-shell-scripting/bash-tutorial/"><span>Bash Scripting</span></a> <a href="/scipy-tutorial/">SciPy</a> <a href="/git/">Git</a> <a href="/opencv/python/"><span>OpenCV Python</span></a> <a href="/javafx/">JavaFX</a> <a href="/uml-tutorial/">UML</a> <a href="/typescript/">TypeScript</a> <a href="/scala/">Scala</a> <a href="/julia/">Julia</a> <a href="/numpy-tutorial/">Numpy</a> <a href="/golang-tutorial/">Golang</a>
Screenshot
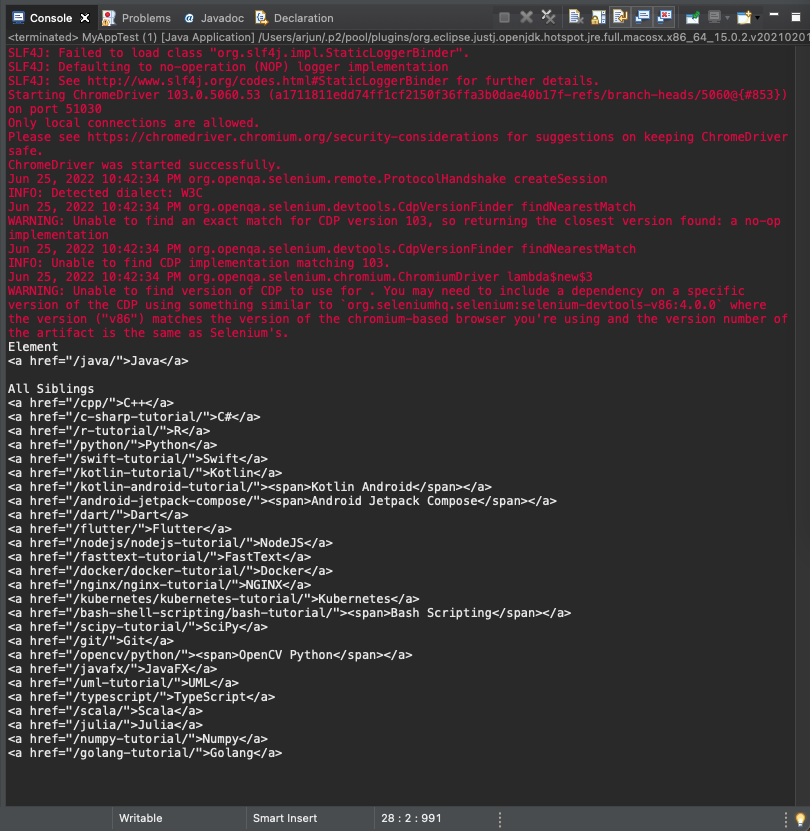