Count Total Number of Web Elements in a Page
To count the total number of web elements in the web page using Selenium for Java, find elements using XPath with expression to match any web element. driver.findElements(By.xpath("//*"))
returns a list containing all the web elements present in the web page. Call size() on this list, and it returns the number of elements in the web page.
The following is a simple code snippet to get the total number of elements in the web page.
List<WebElement> elements = driver.findElements(By.xpath("//*")); int elementsCount = elements.size();
Example
ADVERTISEMENT
In the following program, we write Selenium Java code to visit Tutorialkart Home Page, and get the total number of web elements in the page.
Java Program
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialkart.com/"); List<WebElement> elements = driver.findElements(By.xpath("//*")); int elementsCount = elements.size(); System.out.println("Total Number of Elements : " + elementsCount); driver.quit(); } }
Output
Total Number of Elements : 229
Screenshot
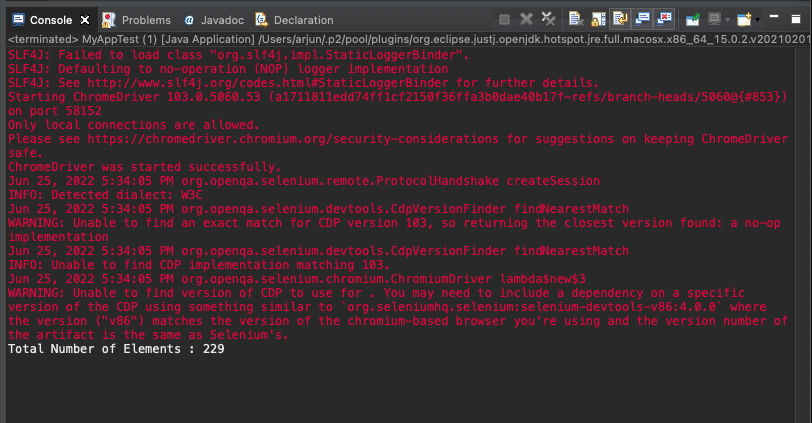