Open Link in New Tab
To open a link in new tab using Selenium in Java, execute JavaScript code to open a new window from the current window using JavaScriptExecutor.executeScript()
, and then we may switch to the new tab using WebDriver.switchTo()
.
The following is a simple code snippet where we open a new tab, switch to the new tab, and then hit a URL in the tab.
</>
Copy
WebDriver driver = new ChromeDriver();
((JavascriptExecutor)driver).executeScript("window.open()");
ArrayList<String> tabs = new ArrayList<String>(driver.getWindowHandles());
driver.switchTo().window(tabs.get(tabs.size()-1));
driver.get("https://www.tutorialkart.com/");
Example
In the following, we write Selenium Java program to visit https://www.google.com/
, then open a new tab, switch to the new tab, and get the URL https://www.tutorialkart.com/
.
Java Program
</>
Copy
import java.util.ArrayList;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
((JavascriptExecutor)driver).executeScript("window.open()");
ArrayList<String> tabs = new ArrayList<String>(driver.getWindowHandles());
driver.switchTo().window(tabs.get(tabs.size()-1));
driver.get("https://www.tutorialkart.com/");
driver.quit();
}
}
Screenshots
1. Initialize web driver and visit https://www.google.com/
.
</>
Copy
WebDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
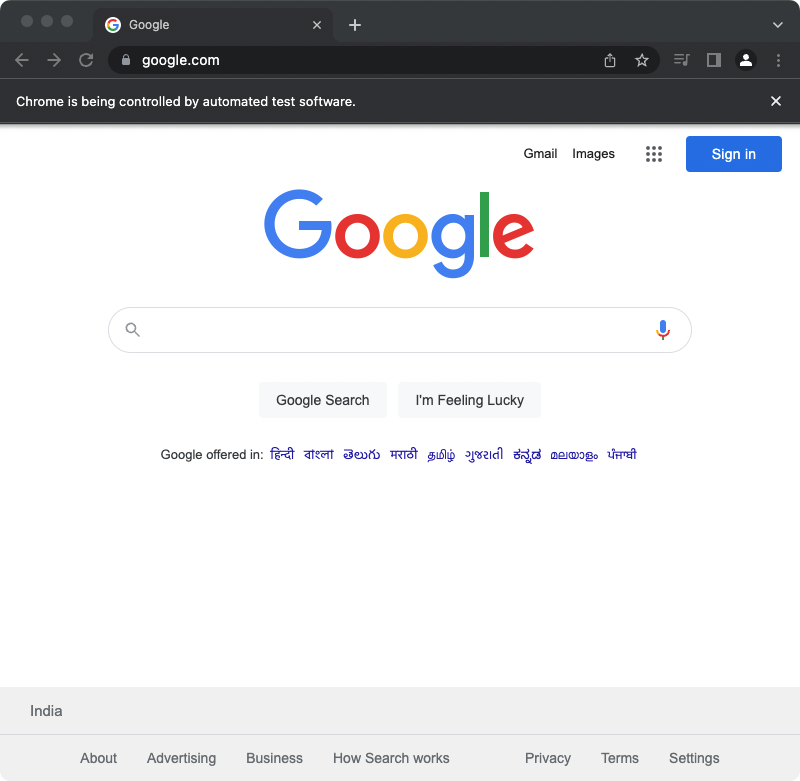
2. Open a new tab and switch to the new tab or last tab.
</>
Copy
((JavascriptExecutor)driver).executeScript("window.open()");
ArrayList<String> tabs = new ArrayList<String>(driver.getWindowHandles());
driver.switchTo().window(tabs.get(tabs.size()-1));
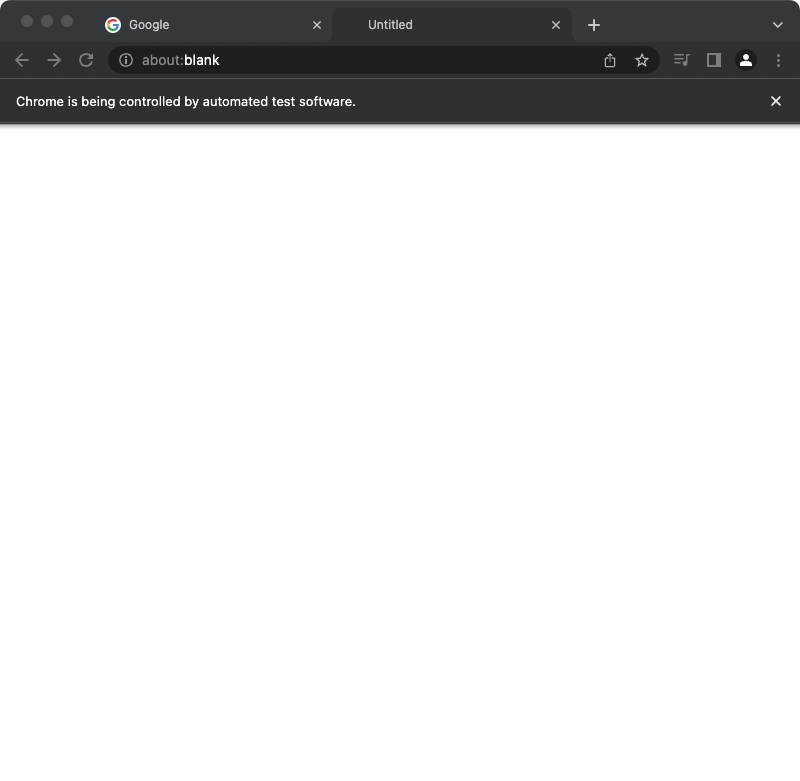
3. Get the URL https://www.tutorialkart.com/
in the new tab.
</>
Copy
driver.get("https://www.tutorialkart.com/");
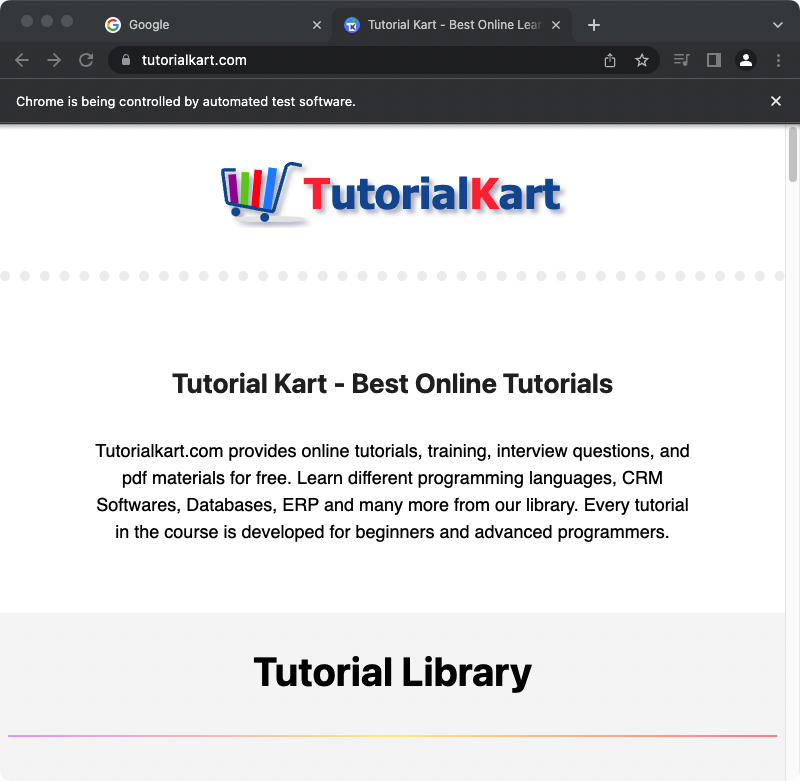