Press Browser’s Back Button
To simulate pressing browser’s back button programmatically using Selenium in Java, call navigate().back()
on the web driver object.
WebDriver.navigate().back()
method simulates a move back a single “item” in the browser’s history.
The following is a quick Java code snippet to press browser’s back button using Selenium.
</>
Copy
WebDriver driver = new ChromeDriver();
//some code
driver.navigate().back();
Example
In the following, we write Java code to initialize a web driver, visit google.com, search for the query 'tutorialkart'
, and press the browser’s back button.
Java Program
</>
Copy
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://google.com/ncr");
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys("tutorialkart");
searchBox.sendKeys(Keys.RETURN);
driver.navigate().back();
driver.quit();
}
}
Screenshots
1. Initialize Web Driver and navigate to google.com.
</>
Copy
WebDriver driver = new ChromeDriver();
driver.get("https://google.com/ncr");
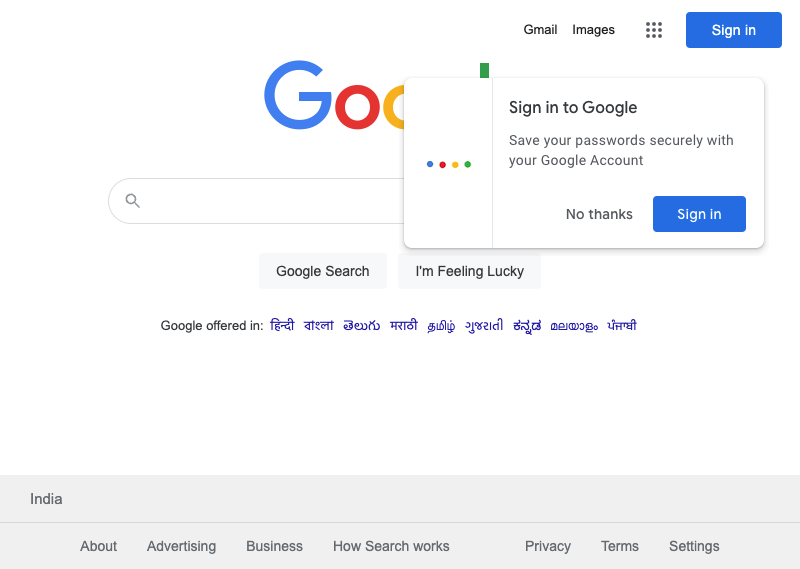
2. Enter serach query: ‘tutorialkart’
</>
Copy
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys("tutorialkart");
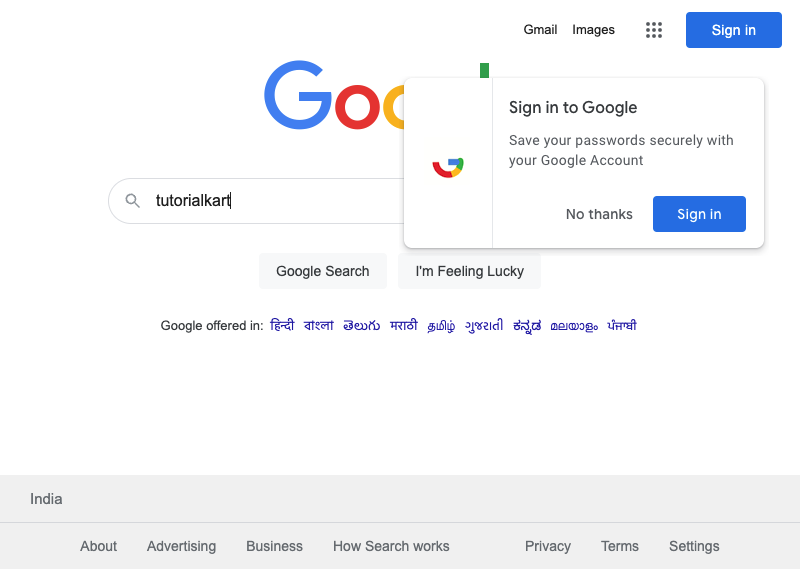
3. Press Enter
</>
Copy
searchBox.sendKeys(Keys.RETURN);
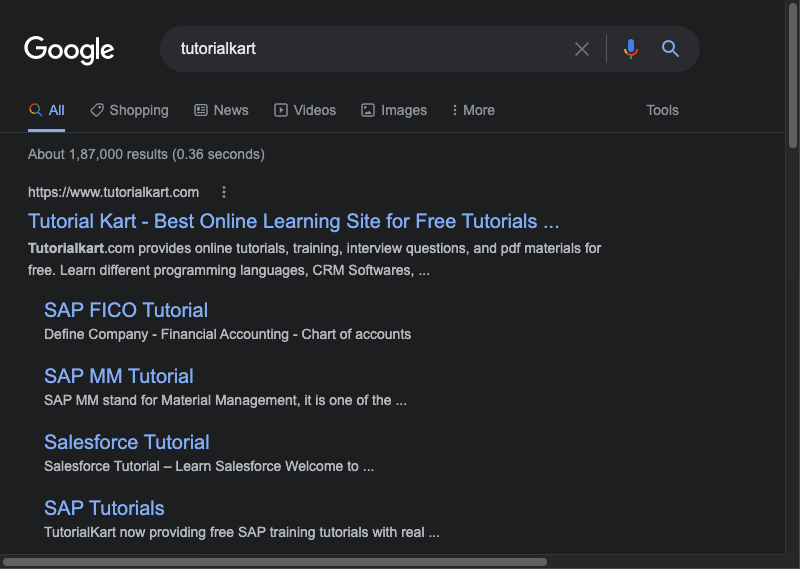
4. Navigate Back
</>
Copy
driver.navigate().back();
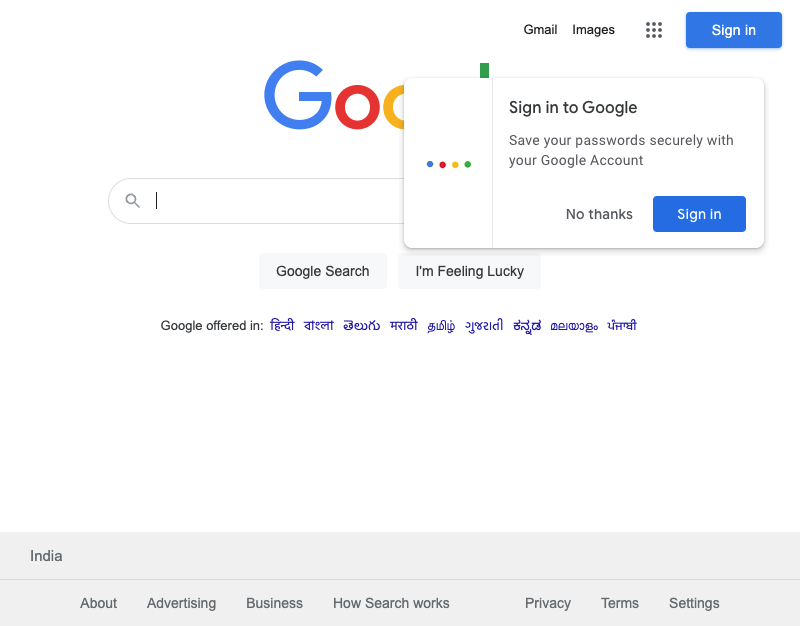